Written by: Ismaël Diakité
Date Published: 28 November 2023
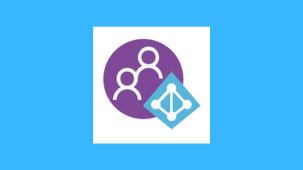
Fetching Azure AD Groups and Users with Microsoft Graph SDK in C#
This tutorial demonstrates how to retrieve groups and users from Azure Active Directory (AAD) using the Microsoft Graph SDK in C#. We'll be using PageIterator
for efficient data retrieval and Parallel.ForEach
for concurrent processing.
Prerequisites
- Azure Active Directory Tenant
- Registered Azure AD Application with Client ID and Secret
- Required API permissions in Azure AD for Microsoft Graph (Group.Read.All and User.Read.All)
- .NET development environment with Azure SDK and Microsoft Graph SDK
Step 1: Setting Up the Environment
First, include the necessary namespaces:
using Azure.Core;
using Azure.Identity;
using Microsoft.Graph;
using Microsoft.Graph.Models;
using System.Collections.Concurrent;
Step 2: Initialize Authentication
To initialize authentication, you need to define the following variables in your code and replace the placeholders with your actual values:
string tenantId = "YOUR TENANT ID HERE";
string clientId = "YOUR CLIENT ID HERE";
string clientSecret = "YOUR CLIENT SECRET HERE";
Step 3: Create a GraphServiceClient
To create a GraphServiceClient
instance using ClientSecretCredential
, follow these steps:
var scopes = new[] { "https://graph.microsoft.com/.default" };
var options = new TokenCredentialOptions
{
AuthorityHost = AzureAuthorityHosts.AzurePublicCloud
};
var clientSecretCredential = new ClientSecretCredential(tenantId, clientId, clientSecret, options);
// Optional: Get access token to verify authentication
var accessToken = await clientSecretCredential.GetTokenAsync(new TokenRequestContext(scopes) { });
Console.WriteLine(accessToken.Token);
var graphClient = new GraphServiceClient(clientSecretCredential, scopes);
Step 4: Retrieve and Process Groups
To retrieve and process groups using the GetGroupsAsync
method and process them in parallel, follow these steps:
var groupsList = await GetGroupsAsync(graphClient);
Parallel.ForEach(groupsList!, group =>
{
Console.WriteLine(group.DisplayName);
});
Step 5: Retrieve and Process Users
To retrieve and process users using the GetUsersAsync
method and process them in parallel, follow these steps:
var usersList = await GetUsersAsync(graphClient);
Parallel.ForEach(usersList!, user =>
{
Console.WriteLine(user.DisplayName);
});
Auxiliary Methods
GetGroupsAsync Method
The GetGroupsAsync
method is designed to fetch all groups from Azure Active Directory (AAD) using a PageIterator
. Below is the code for this method:
async Task<ConcurrentBag<Group>?> GetGroupsAsync(GraphServiceClient graphClient)
{
var groupsList = new ConcurrentBag<Group>();
var requestGroup = graphClient.Groups
.GetAsync()
.GetAwaiter()
.GetResult();
if (requestGroup is null)
{
Console.WriteLine("No group found in Azure AD");
return null;
}
var pageIterator = PageIterator<Group, GroupCollectionResponse>
.CreatePageIterator(graphClient, requestGroup,
group =>
{
groupsList.Add(group);
return true;
});
await pageIterator.IterateAsync();
return groupsList;
}
GetUsersAsync Method
The GetUsersAsync
method is designed to fetch all users from Azure Active Directory (AD) using a PageIterator
. Below is the code for this method:
async Task<ConcurrentBag<User>?> GetUsersAsync(GraphServiceClient graphClient)
{
var usersList = new ConcurrentBag<User>();
var requestUser = graphClient.Users
.GetAsync()
.GetAwaiter()
.GetResult();
if (requestUser is null)
{
Console.WriteLine("No user found in Azure AD");
return null;
}
var pageIterator = PageIterator<User, UserCollectionResponse>
.CreatePageIterator(graphClient, requestUser,
user =>
{
usersList.Add(user);
return true;
});
await pageIterator.IterateAsync();
return usersList;
}
Conclusion
This tutorial demonstrated how to retrieve groups and users from Azure Active Directory (AAD) using the Microsoft Graph SDK in C#. We used PageIterator
for efficient data retrieval and Parallel.ForEach
for concurrent processing.
Full Source Code
The complete source code for this project can be found at the following GitHub repository: FetchGroupsAndUsersAAD